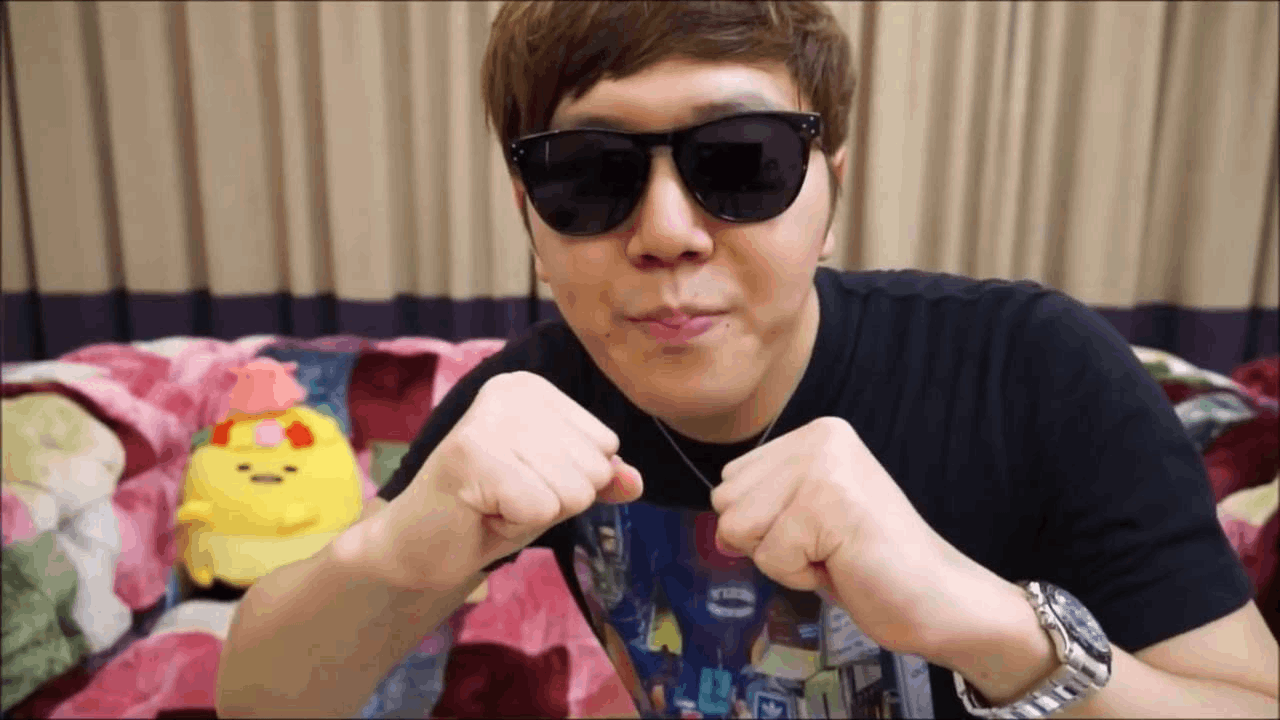
If you're losing to him in terms of annual income and personality,
I want him to at least win rock-paper-scissors.
So, this time,
we will use TensorFlow to create an AI that makes Hikakin Bun Bun win with
an image classifier.
What to prepare
- Google Colaboratory (*Jupyter Notebook is also OK)
- Distribution source code (*Not required if you want to start from scratch)
How to create AI that makes Hikakin Bunbun win
Let AI learn rock-paper-scissors
First, download the zip file containing the rock-paper-scissors image.
!wget --no-check-certificate
https://storage.googleapis.com/laurencemoroney-blog.appspot.com/rps.zip
-O /tmp/rps.zip
!wget --no-check-certificate
https://storage.googleapis.com/laurencemoroney-blog.appspot.com/rps-test-set.zip
-O /tmp/rps-test-set.zip
`
–2022-09-19 05:51:56– https://storage.googleapis.com/laurencemoroney-blog.appspot.com/rps.zip
Resolving storage.googleapis.com (storage.googleapis.com)… 172.217.194.128, 142.251.10.128, 142.251.12.128, …
Connecting to storage.googleapis.com (storage.googleapis.com)|172.217.194.128|:443… connected.
HTTP request sent, awaiting response… 200 OK
Length: 200682221 (191M) [application/zip]
Saving to: ‘/tmp/rps.zip’
/tmp/rps.zip 100%[===================>] 191.38M 40.7MB/s in 4.7s
2022-09-19 05:52:02 (40.7 MB/s) – ‘/tmp/rps.zip’ saved [200682221/200682221]
–2022-09-19 05:52:02– https://storage.googleapis.com/laurencemoroney-blog.appspot.com/rps-test-set.zip
Resolving storage.googleapis.com (storage.googleapis.com)… 172.217.194.128, 142.251.10.128, 142.251.12.128, …
Connecting to storage.googleapis.com (storage.googleapis.com)|172.217.194.128|:443… connected.
HTTP request sent, awaiting response… 200 OK
Length: 29516758 (28M) [application/zip]
Saving to: ‘/tmp/rps-test-set.zip’
/tmp/rps-test-set.z 100%[===================>] 28.15M –.-KB/s in 0.1s
2022-09-19 05:52:02 (204 MB/s) – ‘/tmp/rps-test-set.zip’ saved [29516758/29516758]
`
Unzip the zip and save it in the /tmp/
directory.
import os
import zipfile
local_zip = '/tmp/rps.zip'
zip_ref = zipfile. ZipFile(local_zip, 'r')
zip_ref.extractall('/tmp/')
zip_ref.close()
local_zip = '/tmp/rps-test-set.zip'
zip_ref = zipfile. ZipFile(local_zip, 'r')
zip_ref.extractall('/tmp/')
zip_ref.close()
Categorize rock-paper-scissors images for learning into goo
, choki
, and par
.
rock_dir = os.path.join('/tmp/rps/rock')
paper_dir = os.path.join('/tmp/rps/paper')
scissors_dir = os.path.join('/tmp/rps/scissors')
print('total training rock images:', len(os.listdir(rock_dir)))
print('total training paper images:', len(os.listdir(paper_dir)))
print('total training scissors images:', len(os.listdir(scissors_dir)))
rock_files = os.listdir(rock_dir)
print(rock_files[:10])
paper_files = os.listdir(paper_dir)
print(paper_files[:10])
scissors_files = os.listdir(scissors_dir)
print(scissors_files[:10])
total training rock images: 840 total training paper images: 840 total training scissors images: 840 ['rock01-027.png', 'rock05ck01-108.png', ' rock05ck01-090.png', 'rock01-040.png', 'rock02-108.png', 'rock02-022.png', 'rock01-060.png', 'rock07-k03-061.png', 'rock06ck02-032.png', 'rock03-069.png'] ['paper05-114.png', 'paper03-099.png', 'paper06-011.png', 'paper07-053.png ', 'paper03-040.png', 'paper07-040.png', 'paper05-082.png', 'paper07-116.png', 'paper01-041.png', ' paper07-035.png'] ['scissors04-034.png', 'testscissors01-033.png', 'testscissors03-058.png', 'testscissors01-087.png', ' testscissors03-044.png', 'testscissors01-040.png', 'scissors04-080.png', 'scissors03-069.png', 'testscissors03-088.png', 'scissors04-038.png']
Plot.
%matplotlib inline
import matplotlib.pyplot as plt
import matplotlib.image as mpimg
pic_index = 2
next_rock = [os.path.join(rock_dir, fname)
for fname in rock_files[pic_index-2:pic_index]]
next_paper = [os.path.join(paper_dir, fname)
for fname in paper_files[pic_index-2:pic_index]]
next_scissors = [os.path.join(scissors_dir, fname)
for fname in scissors_files[pic_index-2:pic_index]]
for i, img_path in enumerate(next_rock+next_paper+next_scissors):
img = mpimg.imread(img_path)
plt.imshow(img)
plt.axis('Off')
plt.show()
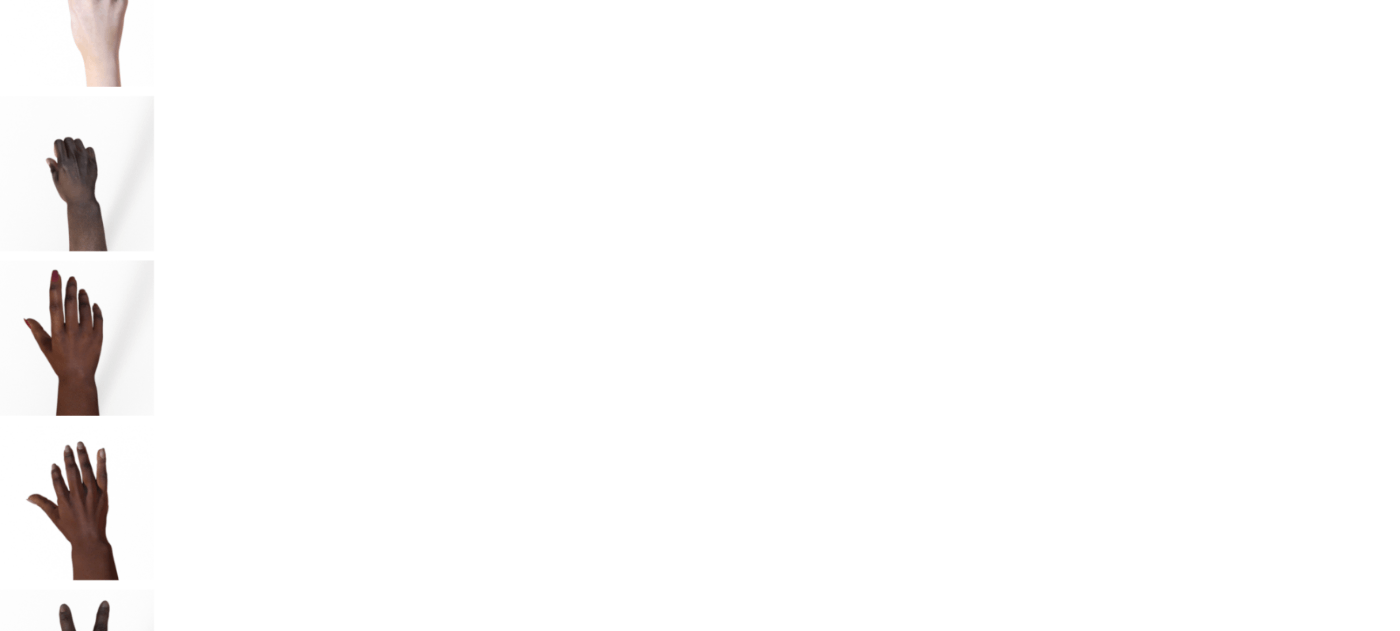
Learn.
import tensorflow as tf
import keras_preprocessing
from keras_preprocessing import image
from keras_preprocessing.image import ImageDataGenerator
TRAINING_DIR = "/tmp/rps/"
training_datagen = ImageDataGenerator(
rescale = 1./255,
rotation_range=40,
width_shift_range=0.2,
height_shift_range=0.2,
shear_range=0.2,
zoom_range=0.2,
horizontal_flip=True,
fill_mode='nearest')
VALIDATION_DIR = "/tmp/rps-test-set/"
validation_datagen = ImageDataGenerator(rescale = 1./255)
# Create training data
train_generator = training_datagen.flow_from_directory(
TRAINING_DIR,
target_size=(150,150),
class_mode='categorical',
batch_size=126
)
# Create verification data
validation_generator = validation_datagen.flow_from_directory(
VALIDATION_DIR,
target_size=(150,150),
class_mode='categorical',
batch_size=126
)
# Define a neural network
model = tf.keras.models.Sequential([
tf.keras.layers.Conv2D(64, (3,3), activation='relu', input_shape=(150, 150, 3)),
tf.keras.layers.MaxPooling2D(2, 2),
tf.keras.layers.Conv2D(64, (3,3), activation='relu'),
tf.keras.layers.MaxPooling2D(2,2),
tf.keras.layers.Conv2D(128, (3,3), activation='relu'),
tf.keras.layers.MaxPooling2D(2,2),
tf.keras.layers.Conv2D(128, (3,3), activation='relu'),
tf.keras.layers.MaxPooling2D(2,2),
tf.keras.layers.Flatten(),
tf.keras.layers.Dropout(0.5),
tf.keras.layers.Dense(512, activation='relu'),
tf.keras.layers.Dense(3, activation='softmax')
])
# Summary
model.summary()
# Compiling the neural network
model.compile(loss = 'categorical_crossentropy', optimizer='rmsprop', metrics=['accuracy'])
# Learn
history = model.fit(train_generator, epochs=25, steps_per_epoch=20, validation_data = validation_generator, verbose = 1, validation_steps=3)
model.save("rps.h5")
The study is over.
Play rock-paper-scissors with Hikakin and let the AI win
Now upload the file and output the winning hand to Hikakin.
import numpy as np
from google.colab import files
from keras.preprocessing import image
uploaded = files.upload()
for fn in uploaded.keys():
path = fn
img = image.load_img(path, target_size=(150, 150))
x = image.img_to_array(img)
x = np.expand_dims(x, axis=0)
images = np.vstack([x])
classes = model.predict(images, batch_size=10)
hikakin_result = np.array(classes[0])
paper = np.array([1, 0, 0])
rock = np.array([0, 1, 0])
scissors = np.array([0, 0, 1])
if hikakin_result[0] == paper[0]:
print("✌")
if hikakin_result[1] == rock[1]:
print("🖐")
if hikakin_result[2] == scissors[2]:
print("✊")
Let's do the above.
I will try to play rock-paper-scissors in the 10th round.
Round 1
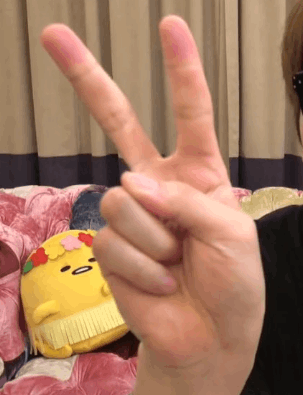

✊ AI put out.
Therefore, Round 1 is won by AI.
Round 2
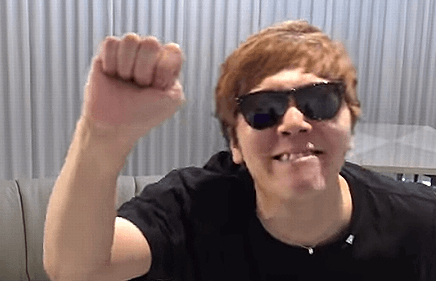

🖐 AI put out.
Therefore, Round 2 is a win for AI.
Round 3
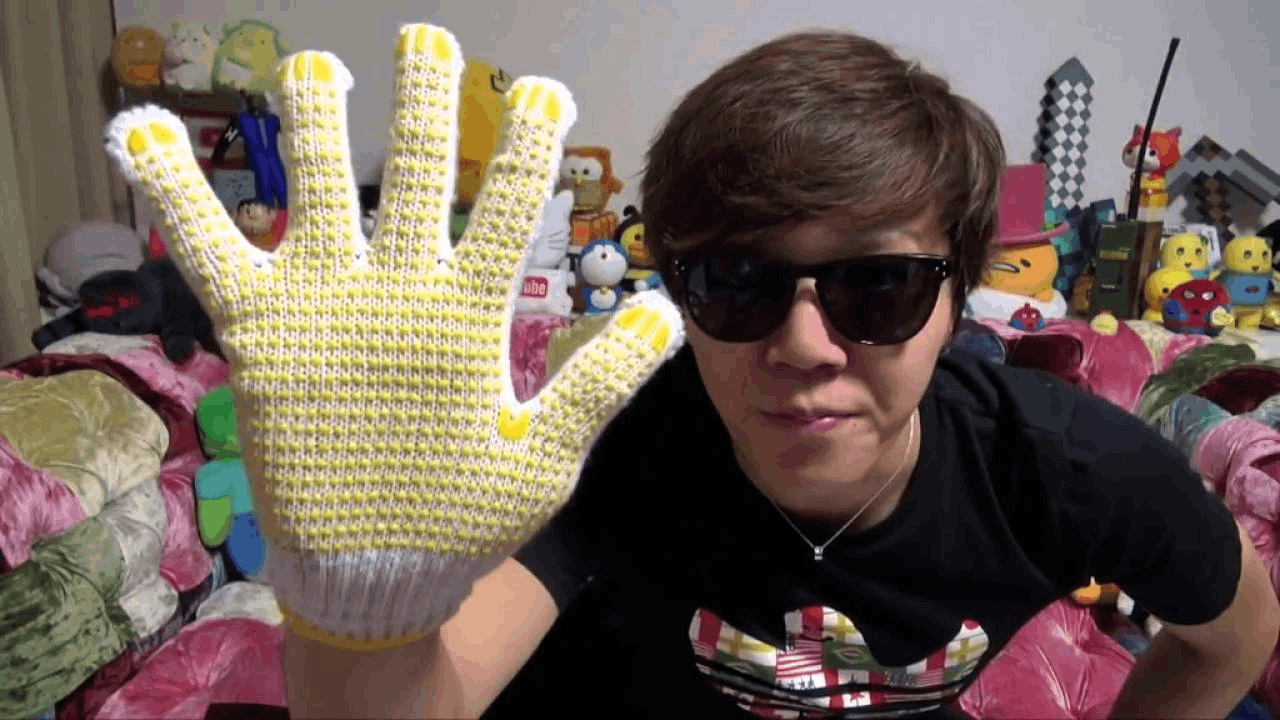

🖐 AI put out.
Therefore, Round 3 is Aiko.
Round 4
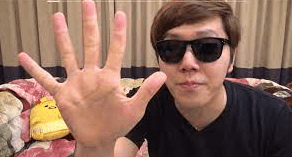

✊ AI put out.
Therefore, Round 4 is a loss for AI.
Is AI weak when parred?
Round 5
Will you be honest for the third time?
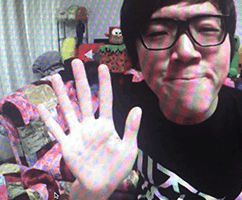

✌ AI put out.
Therefore, Round 5 is a win for AI.
Finally, I was able to win par.
Round 6
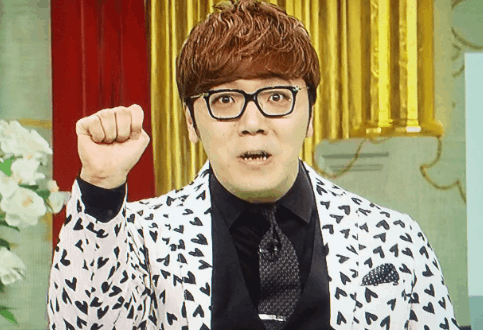

🖐 AI put out.
Therefore, Round 6 is a victory for AI.
Round 7
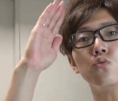

✌ AI put out.
Therefore, Round 7 is a win for AI.
Round 8
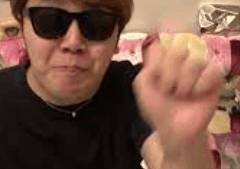

✌ AI put out.
Therefore, Round 8 is a loss for AI.
It was useless if it was shaky.
Round 9
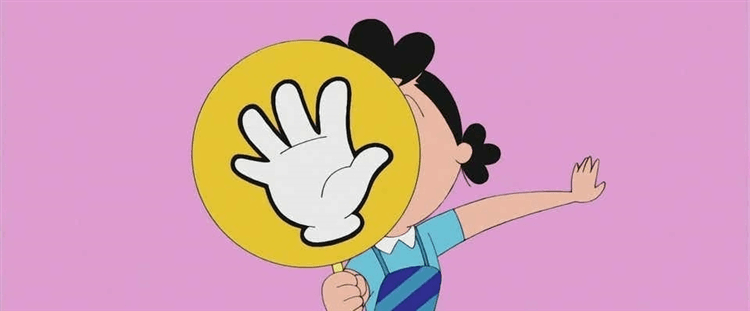

✌ AI put out.
Therefore, Round 9 is a win for AI.
Round 10
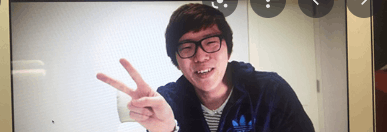

🖐 AI put out.
Therefore, Round 10 is a loss for AI.
Results
Round | AI | Hikakin |
---|---|---|
1 | Yes | |
2 | Yes | |
3 | – | – |
4 | Yes | |
5 | Yes | |
6 | Yes | |
7 | Yes | |
8 | Yes | |
Nine. | Yes | |
10 | Yes |
AI can beat rock-paper-scissors 70% of the time
Click here for DeepTech's magazine "DeepMagazine"